W01 Assignment: Course Home Page
Overview
This assignment demonstrates your prerequisite knowledge by applying HTML, CSS, and JavaScript to the design and development a course home page.
Task
Design and develop the a course home page using the following wireframe as a guide for the layout and content. The page must be responsive and pass performance, accessibility, best practice, and SEO tests.
Screenshots
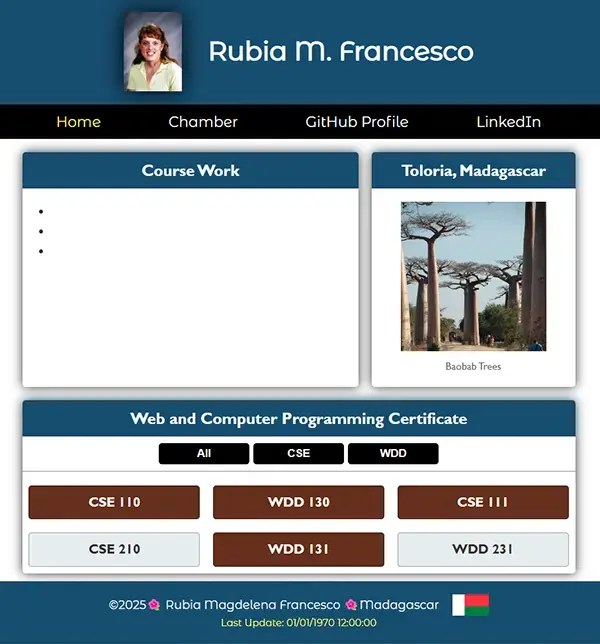
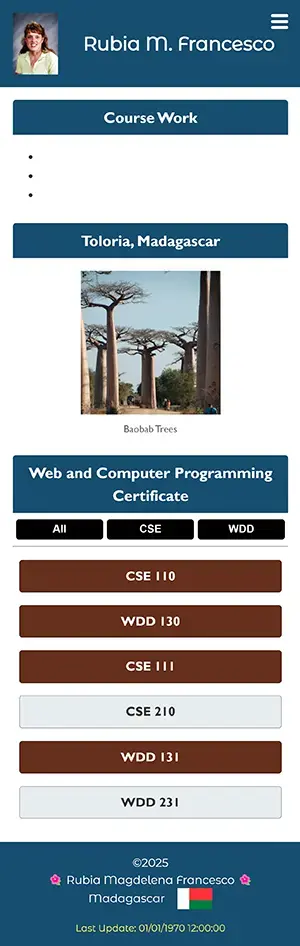
Instructions
File and Folder Setup
- In VS Code, open your
wdd231
folder that you cloned from your GitHub account during the Setup: Hosting on GitHub activity. - Create a default home page for this repository so that when published to GitHub, will render at
https://yourgithubusername.github.io/wdd231
. What must this file be named?Check Your Understanding
index.html
- Create appropriately named, supporting folders for images, CSS, and JavaScript files.
These sub-folders must be placed in the root directory,wdd231
.Check Your Understanding
- Images will be stored in a folder named
images
- CSS files will be stored in a folder named
styles
- JavaScript files will be stored in a folder named
scripts
Note that the words directory and folder have essentially the same meaning. Directory is the more accurate term for file systems while "folder" 📂 refers to the graphical metaphor that is generally accepted because it is highly related to the term "file" in the organized world.
- Images will be stored in a folder named
- Add CSS files as needed.
Check Your Understanding
Students are required to write their own custom CSS in this course. Frameworks are not allowed at this point.
The assignment requires you to create a responsive design.
You may elect to use a single CSS file or multiple CSS files. - Add a JS file references as needed. For example, create files to support the responsive navigation, dates, and
course information cards (see below). Reference these in the
head
using thedefer
attribute method.
HTML
- Add the standard HTML document structure to the document.
- Add the required elements to the
<head>
including thetitle
,meta description
, and themeta author
. - Use semantic HTML to create the basic layout structure of the page.
Consider using aheader
, anav
, amain
, and afooter
element. - Add the content as shown in the example screenshots above.
- Note that all images used on your pages must be optimized.
In this class, the image memory threshold is 125 kB or less per image.
- The responsive navigation
<nav>
menu contains four links:- Home - this current page.
- Chamber - will link to the Chamber of Commerce website project (future assignment).
- GitHub Profile - will link to your GitHub Profile page (future assignment).
- LinkedIn - links to your LinkedIn profile page.
If you do not have a free LinkedIn account profile, consider making one or just provide a general link to LinkedIn.
- The
<main>
element contains the following:- Three
<section>
tags with headings<h2>
as shown in the example screenshots above.
- Three
- The
<footer>
has two paragraphs<p>
.- The first paragraph contains the following:
- the copyright symbol and current year that are written dynamically using JavaScript
Check Your Understanding
In HTML, provide a placeholder that can easily be selected using JavaScript.
Example:
<span id="currentyear"></span>
- your name
- your state or country
- the copyright symbol and current year that are written dynamically using JavaScript
- The second paragraph has an id of "lastModified" and will be populated with JavaScript code.
- The first paragraph contains the following:
CSS
- Use your own color schema and typography choices.
You are responsible to practice good design principles including alignment, color contrast, proximity, use of white space, repetition, and typography.
- Use the Google Fonts API
to select one or two fonts to use on the page.
Video Demonstration: ▶️ Using Google Fonts
- Responsive navigation must, at least, support the following features:
- small-screen hamburger like navigation
- large-screen horizontal navigation using CSS
Flex
Video Demonstration: ▶️ CSS Flex Navigation Menu
Source code: CSS Flex Menu - CodePen - wayfinding
Wayfinding supports user friendly navigation by providing a clear indication of the user's current location within a website.
Here is an example of designing wayfinding into a navigation menu: Responsive Menu.
- hover effect for menu items
Responsive design includes providing a positive user experience of readability, usability, accessibility, visual appeal that adapts to the user's device by size, orientation, and resolution.
- Your design cannot just be a simple one column layout (default flow layout) in all views in order to warrant full credit consideration.
JavaScript
- Reference JavaScript files by using a
<script>
reference in thehead
of the HTML file and using the attributedefer
.Why is the defer boolean attribute important?
- Use JavaScript to support the responsive menu.
- Use JavaScript to dynamically output the following:
- the copyright year (the current year) in the footer's first paragraph
Note this CodePen summary of the
Date
object. - the date the document was last modified in the second paragraph
You can use the lastModified property of the document object to get this date/time dynamically.
Note that the
document.lastModified
returns a simple string. Therefore, you do not need to manipulate its output.
- the copyright year (the current year) in the footer's first paragraph
- Copy this array of course objects into a JavaScript file: Course List Array
This array contains the course information for the required courses that are in the first certificate called Web and Computer Programming of the Software Development degree.
- Modify the courses array content in your script file by changing the
completed
property totrue
if you have completed a course. - Dynamically display all the courses in the certificate section as shown in the example above. The courses that you have completed must be marked in a different way versus those that you have not completed. Use your page color scheme. The page should adjust automatically if the data source changes.
- Using buttons, allow the user to select to display all courses, WDD courses, or CSE courses. Use the array
(
filter
) method.Course Filter Buttons Demonstration
- Design the course cards to indicate those courses that you have completed in a complimentary, but different style than the rest.
- Provide a total number of credits required dynamically by using a
reduce
function (not shown on the screenshots). The number of credits shown should reflect just the courses currently being displayed.
Testing
- Continuously check your work by having it loaded in your browser using Local Server.
-
"DevTools" is an abbreviation for the browser's "Developer Tools." It refers to a set of tools or utilities provided by web browsers to help developers debug, profile, and analyze web pages during the development process. The tools are typically accessed by pressing the F12 function key or selecting the menu option for the browser's developer tools.
- Use DevTools CSS Overview to check your color contrast.
- Generate the DevTools
Lighthouse
report and run diagnostics for
Performance,
Accessibility,
Best Practices, and
SEO
in both the mobile and Desktop views.
Use the Private or Incognito mode to test your page when using Lighthouse. The standard is to reach a score of 90 or better in each of these categories.
Submission and Audit
- Commit and sync your local work to your a GitHub Pages enabled wdd231 repository
- Use this ☑️ audit tool to self-check your work for some of the required HTML elements and CSS content. This audit tool is also used by the assessment team.
- Return to I-Learn and submit your GitHub Pages enabled URL for wdd231, e.g.,
https://your-github-username.github.io/wdd231
💡Note that we do not need to include index.html in our reference because index.html is the default file retrieved with a folder request.