W05: Team Activity - JSON and the Weather API
Overview
This activity will have you demonstrate your understanding of the learning activities by applying the concepts to your home page. Work with your team to apply the following specifications to enhance your own, course home page.
Work with your team on applying these concepts from the learning
activities and submitting your own, updated page for feedback and assessment.
Instructions
Dynamic Weather Information
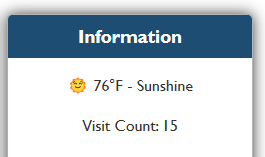
- Open up your individual index.html, course home pages.
- Using openweathermap api, add the current weather conditions to the
information card that currently has placeholder values. At the least, your information card
should contain the following:
- The current temperature.
- The description of the current condition(s).
- The current condition weather icon.
Remember that the Weather API learning activity provides an example of how to complete this task.
Dynamic Activity Links
This part of the assignment has you change your static learning activity links on your home page to a dynamic list of links using a JSON data source.
JSON Data
- Create a new folder named "data" within your wdd230 root folder.
- In that new
data
folder, create a new JSON file named "links.json" - In the
links.json
file, add a single array of objects named "weeks" - Add two properties to the first array object named "week" and "links".
- The
week
property value should contain the week number as a string written out like this: "Week 1" - The
links
property contains an array of objects with two properties "url" and "title". - The
url
property value contains the relative address to the learning activity file. - The
title
property value contains a short descriptive name of the learning activity. - Add addition
weeks
array values in the same way for weeks 2 through 5.
Example
{
"weeks": [
{
"week": "Week 1",
"links": [
{
"url":"week01/holygrail.html",
"title":"HG Layout"
},
{
"url":"week01/media-query.html",
"title":"mQueries"
}
]
},
...
JavaScript - Build Link Menu Dynamically
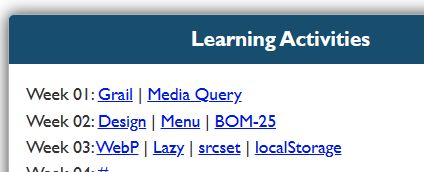
Use JavaScript and this new JSON source file to replace the static learning activity links with dynamically built list of weeks and activity links.
The rendered learning activity section will still look the same. You
will replace all the content with a blank element, ready to be built with a list of week
numbers and their associated hyperlinks using JavaScript.
- In your scripts folder within the wdd230 root folder, add a new JavaScript file named "links.js".
- In the links.js file, create a baseURL variable that references your root
wdd230 repository, GitHub pages URL.
Example
const baseURL = "https://yourgithubusername.github.io/wdd230/";
- Next, add a variable named linksURL that references your links.json data
file.
Example
const linksURL = "https://yourgithubusername.github.io/wdd230/data/links.json";
- Write an asynchronous function to get the links data in the links.json data file.
- Test the JSON result by writing the data to the console. In order to test your
work at this point, you will need to write a line of code in links.js which calls the
getLinks() function. Once your are satisfied or producing links, remove the
console.log() statement.
Example
async function getLinks() { const response = await fetch(linksURL); const data = await response.json(); console.log(data); } getLinks();
- In the getLinks function, add a new line of code at the end that calls a function that
will build out the available activity links from the data response.
- Name the function displayLinks.
- Send the data as an argument.
Example
async function getLinks() { const response = await fetch(linksURL); const data = await response.json(); displayLinks(data); }
- Create the displayLinks() function and name the function's single parameter weeks. Remember from the json data that you wrote and tested that the data is an array of objects representing weeks of the term.
- Using your existing static HTML links structure in your index.html document as a guide,
use a loop to process each week using it's "week" string and "links' object ("url" and
"title") to create the output.
The latter-day prophets application in the learning activity provided you with an example to follow.
Testing
- Always practice good design and development techniques by adhering to the course development standards.
Submission
- Commit your local repository and push your work to your wdd230 GitHub Pages enabled repository on GitHub.
- Submit your home page URL in I-Learn.