W04 Activity: Setup a Local Test Environment
Overview
Testers are often required to test pre-release versions of software on a dedicated testing environment. Sometimes, that testing environment is created on your own computer! In this exercise we will set up your computer to run the latest version of the Teton Chamber of Commerce Web Site so you can do an integration test. In order to set up your test environment you need to understand how the Teton Chamber of Commerce system is designed.
System Overview
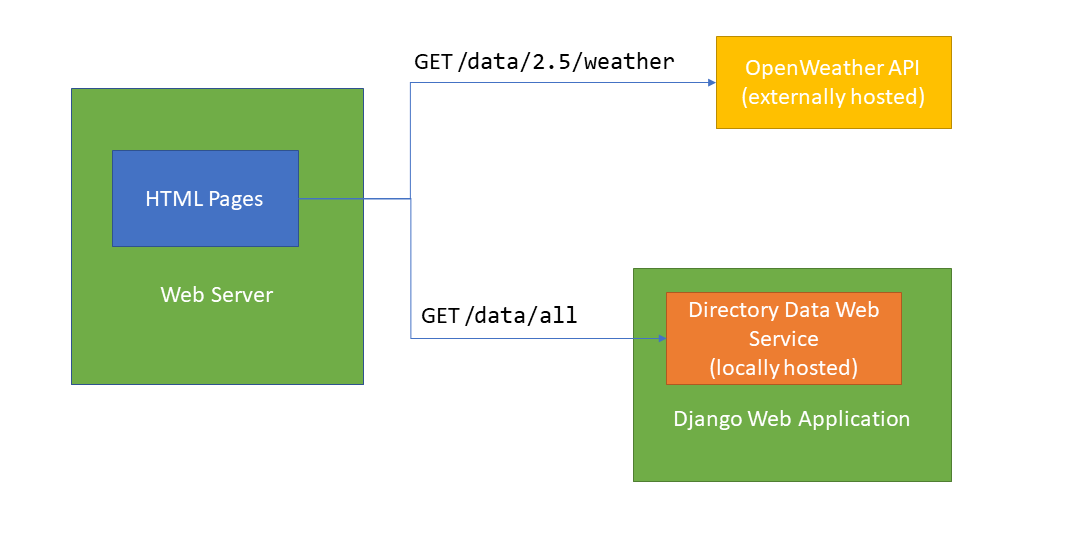
The Teton Idaho Chamber of Commerce Web Site is a vanilla HTML/CSS/Javascript application that gets source data from two primary sources.
Weather data comes from an API call to OpenWeather API. Information about how the API works is found at https://openweathermap.org/current
Directory data comes from an API call to a service that development team wrote. The service was written in Python using the Django Web Application Framework. The web service has a single endpoint /data/all that returns data for all members in the database.
HTTP Method | URL | Sample Response |
GET | /data/all |
|
Setting Up A Local Environment
In order for the application to run independently on your computer, you will need to set up a web server that can display the web pages of the Teton Chamber of Commerce application. You will also need to setup a Django Application Server to run the Directory Data Service.
The OpenWeather API is hosted externally which gives us a couple of options. If it's safe to call the third party production environment as part of your test, then you can do that. Often times, it's not safe to do that and the third party system will ask you to call a separate endpoint for testing purposes. In this instance, there is not a separate test environment, so we can test against the production URL for the weather API.
Installing a Local Web Server (LiveServer)
The integrated development environment VS Code comes with an extension called Live Server which we can use run our web site locally. In many cases you will already have this installed on your computer from previous courses. If you have not yet installed VS Code, navigate to the link below and install the software for your platform.
https://code.visualstudio.com/
Once VS Code has been installed you must install the Live Server using the extensions installer.
- In the menu on the left side, click the extensions icon.
- Next, type "Live Server" in the search box and press Enter or Return.
- Select the Live Server item in the search results.
- Click the "Install" button on the right hand side.
-
Review to the diagram below for an example.
Live Server Extension
Now, open the cse270 folder you downloaded from the main instructions in VS Code. Your VS Code will look like this, but with the correct website version folder:
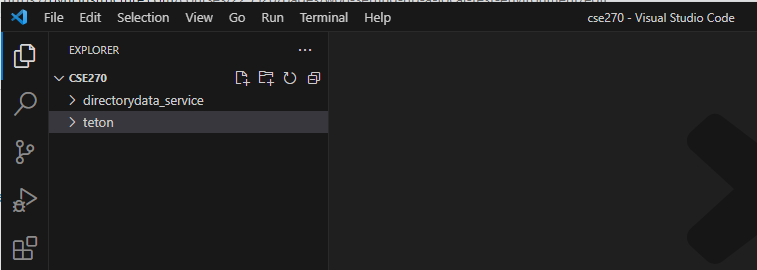
You are now ready for the next steps.
Installing Python and Django
The Directory Data Service requires the installation of Python and Django. Many of you will have already installed Python previously. If you have not, or it has been more than a year since you did, navigate to the link below and install the latest version of Python for your platform.
After downloading the executable file, if you are on Windows, make sure you right-click and "Run as administrator". This avoids errors later.
https://www.python.org/downloads/
Follow the installation prompts.
To ensure python is running correctly, from VS Code choose Terminal then
New Terminal. This is found by choosing View ==> Terminal from the menu.
On Windows at the command line in the terminal type the following command
py --version
On Mac the command is slightly different
python3 --version
If those don't work, you can also try:
python --version
The py command may be substituted for python in all other situations if that is what works.
The version of python currently installed should print in the terminal window similar to below. If it does not, try re-installing python and make sure that the installer adds python to your PATH. If you are still having trouble, reach out to your instructor for help. Do not proceed to the next step until this is working .
Next, in the same terminal window type the following command to install the Django web framework.
On Windows use the following command:
pip install django
On Mac use the following command:
pip3 install django
If you receive an error that "pip is not recognized", use one of these commands instead.
py -m pip install django
python -m pip install django
This will install several packages to enable Django to work on your local machine. After installation, type the command below to ensure Django is working.
django-admin --version
This may result in an error. If it does, you can try one of these two options:
py -m django-admin --version
python -m django-admin --version
If all is well you will see the version of Django installed on your system printed out to the console as below.
OR
Follow the steps below for starting the Directory Service.
Starting the Data Directory Service
With all of the prerequisites installed, you are now ready to start the data directory service.
Open a new terminal in VS Code or reuse the terminal you already have open.
Change to the
datadirectory_service folder and start the server by typing (or copying/pasting) the following:
On Windows:
cd directorydata_service
python manage.py runserver
On Mac:
cd directorydata_service
python3 manage.py runserver
If everything is working you should see the following output which indicates that the server has started locally on port 8000. This means Django installed correctly as well. You can ignore the error about unapplied migrations.
DO NOT click on the "http://127.0.0.1:8000"! The Directory Service has no UI and is not the website you will test. Follow the instructions below for starting the web server to see the Teton Chamber of Commerce page.
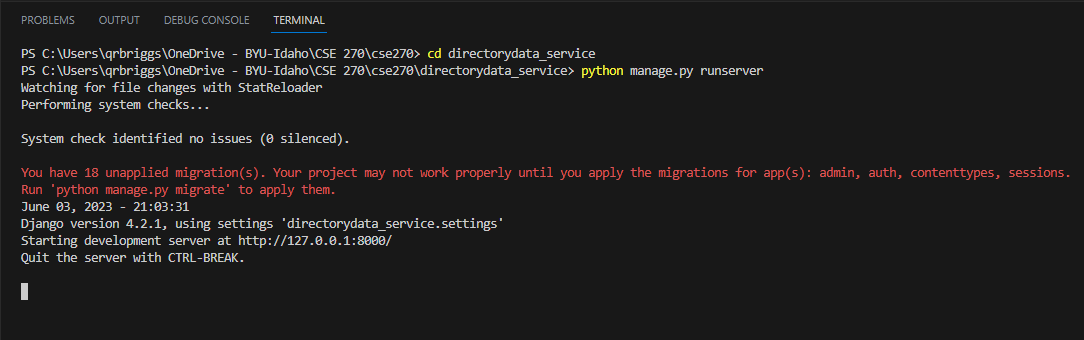
Starting the Web Server
In VS Code, open the teton/1.2 folder and choose the index.html file.
With the index.html file selected, click the "Go Live" icon in the bottom right corner of VS Code.
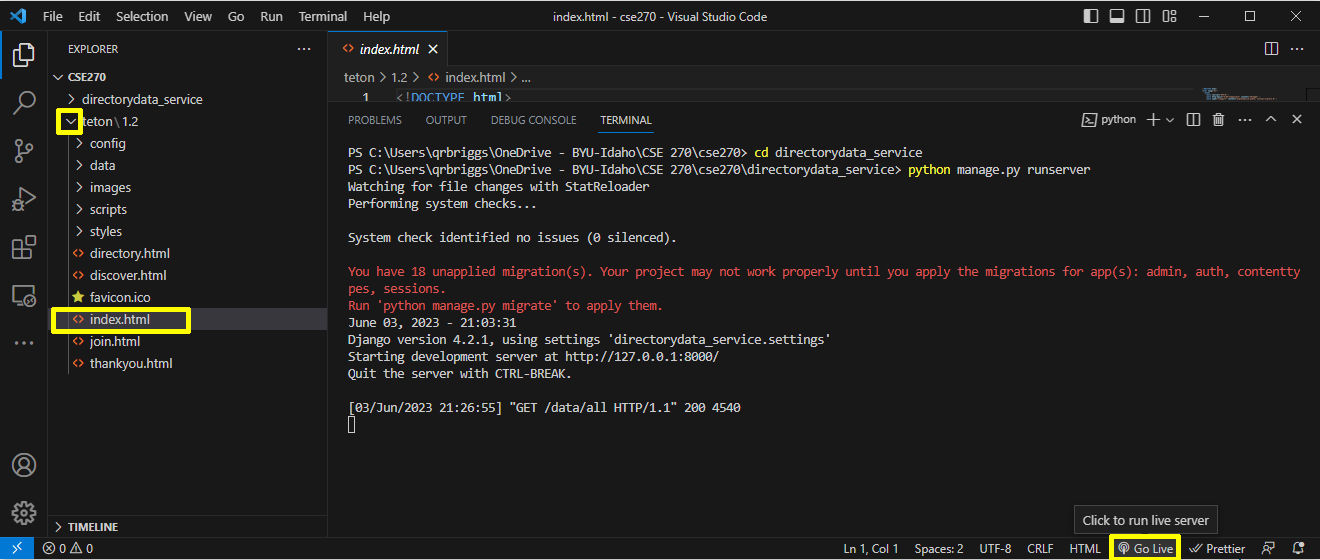
Doing this will open the Teton Chamber of Commerce Home Page in your default browser. If you look things over, both the weather and the spotlights should be present on the page, meaning everything worked as it should. You are now ready to conduct your integration test.
Updating the Configuration File
It's common practice for configuration files to be part of the development process. In this example, the website configuration file lives in the file teton/1.2/config/config.js. This allows testers to update the configuration of the system while doing their testing. Typically, a CI/CD server updates the configuration one last time before deployment with values that are appropriate for the production system.
As previously explained, there is no test environment for the Weather API. Right now, the system is set up to point to a stub. A stub is a placeholder that contains data typical of a valid response from the server that can be used until the "real" system is built. Because we need to test the system against the live data, we need to make two edits to this configuration file.
- To get the api key needed for weather you will need to complete the Installing and Using Postman section of the W04 Activity: Integration Testing Tools and Techniques . Use this API key for the weather API. Update the value in line 3, replacing the text <PROVIDE_KEY_HERE> with the API Key you generated.
- In line 8, change the apiURL value to WEATHER_URL_PROD instead of WEATHER_URL_STUB.
The directory data is also pointing to a stub. In this instance, we do have a test server for the directory data.
- In line 14, change the businessDataUrl value to DIRECTORY_DATA_URL_TEST instead of DIRECTORY_DATA_URL_STUB.
The environment is now set up for testing.
Conclusion
Setting up a local test environment manually can be time consuming and complicated. Many technical workplaces have now automated the setup of test environment using tools like Docker and Kubernetes. It's still very common for test engineers to set up testing environment on their local machines to do testing, and this gives you just a taste of what this might look like.
Submission
Useful Links:
- Return to: Week Overview | Course Home | Canvas