JavaScript: DOM Manipulation
Overview
One of the key skill sets of any frontend web developer is the ability to manipulate the document content using the DOM. The DOM is the outline of the HTML and content nodes. The purpose of this activity is to strengthen our understanding of the DOM and to change or manipulate the document using JavaScript. Learning JavaScript establishes a strong foundation upon which to build, regardless of any frontend JavaScript framework evolutions.
Prepare
- Read: ๐ Manipulating documents - MDN.
Note that the ๐ Active learning: A dynamic shopping list example found at the end of the MDN article will help you complete this activity.
- Review: Common DOM manipulation concepts/use cases.
- To select an HTML element from the document - using the querySelector method.
This line of code selects the first instance of an article element from the document and assigns the element as a reference to the variable namedarticle
.const article = document.querySelector('article');
- To change the innerHTML property of an existing element.
This line of code uses an existing variable that has already selected an element and changes its innerHTML property value.article.innerHTML = 'innerHTML supports <strong>HTML</strong> tags. The textContent property does not.';
- To change the inline CSS style of an element.
This line of code changes the text-align CSS property of the selected element.article.style.textAlign = 'right';
- To change an attribute of an element.
This line of code adds and attribute class to the element and gives it a value.article.setAttribute('class', 'active');
- To create an element.
This line of code creates a new <p> element and stores it in the variable named paragraph.const paragraph = document.createElement('p');
- To append an element with additional content or elements.
These lines of code add content to the end of the article element.article.appendChild(paragraph); article.append('Hello World Addition!');
- To remove an element from the document.
These lines of code remove the paragraph from the article and then, the article itself.article.removeChild(paragraph); article.remove();
- To select an HTML element from the document - using the querySelector method.
Activity Instructions
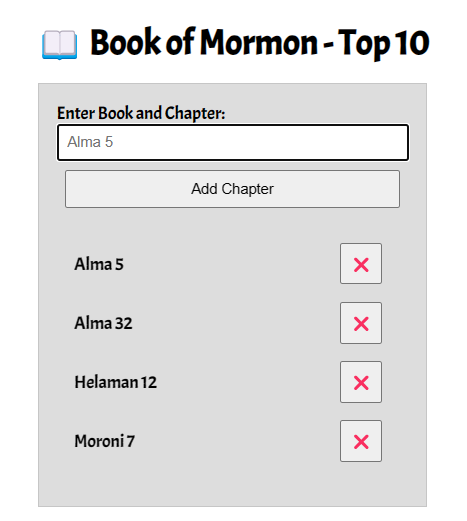
Build the following application which allows users to enter their favorite Book of Mormon chapters and display them on a list that is updated automatically on the screen. Entries can be deleted from the displayed list by the user.
- Create an HTML file named "bom.html" in your week02 folder.
- Your HTML document should include the basic meta tags and a title.
- Create an external CSS file and a JS file and place them in appropriate sub-folders within the week02 folder.
- Use the following โ๏ธCodePen that provides the basic HTML structure of the input and output areas of the app along with some basic CSS: BOM Top 10
- In your js file, declare three const variables that hold references to the
input, button, and .list elements.
Example
const input = document.querySelector('#favchap'); const button = document.querySelector('button'); const list = document.querySelector('#list');
- Create a click event listener for the Add Chapter button using
addEventListener and an anonymous function or arrow function.
Examples
button.addEventListener('click', function() { ... });
button.addEventListener('click', () => { ... });
The ... represents a placeholder for lines of code. Be careful with your opening and closing brackets.
- In the click event function block {...}, do the following:
- check to make sure the input is not blank before
doing the following remaining tasks in this list using an if block,
otherwise provide a message or at least do nothing and return the
.focus() to the input field.
Example
if (input.value != '') { ... }
This example just checks if the input is not blank with no else branch. It just does not do anything the field is blank. The else branch could contain a output statement to a message that reminds the user to enter a book and chapter. There are many ways this could be done. All of the following code would go in the true branch { ... }.
- create a li element
Example
const li = document.createElement('li');
- create a delete button
Example
const deleteButton = document.createElement('button');
- populate the li elements textContent or innerHTML with the input value
Example
li.textContent = input.value;
- populate the button textContent with a โ
Example
deleteButton.textContent = 'โ';
- append the li element with the delete button
Example
li.append(deleteButton);
- append the li element to the unordered list in your HTML
Example
list.append(li);
- add an event listener to the delete button that removes the li element when clicked
Example
deleteButton.addEventListener('click', function () { list.removeChild(li); input.focus(); });
- send the focus to the input element
Example
input.focus();
- change the input value to nothing or the empty string to clean up the interface for
the user
Example
input.value = '';
- check to make sure the input is not blank before
doing the following remaining tasks in this list using an if block,
otherwise provide a message or at least do nothing and return the
.focus() to the input field.
- Test the functionality of your application in your localhost. Resolve errors by using the DevTools in your browser.
Submission
- Update your home portal page to include a link to this application.
- Commit your work and push it up to your GitHub Pages wdd230 repository.
Additional Resources
- ๐ฆ DOM Manipulation – by Web Dev Simplified – 14 key techniques in summary demonstration format. Take special note of the concept of datasets.
- We do need to consider screen readers and how they will interpret anything that we have in content. For example, the delete button just has an emoticon and may not read correctly as the button to remove a chapter. What can we do? One solution is to create a aria-label attribute on the button with a value like "Remove Alma 5"