W05 Assignment: Product Review Form
Overview
In this assignment, you will create product review form page. You will use good form design, be given specific field requirements, and you must include a submission confirmation with a counter. In addition, the form must be user friendly on both mobile and desktop screens.
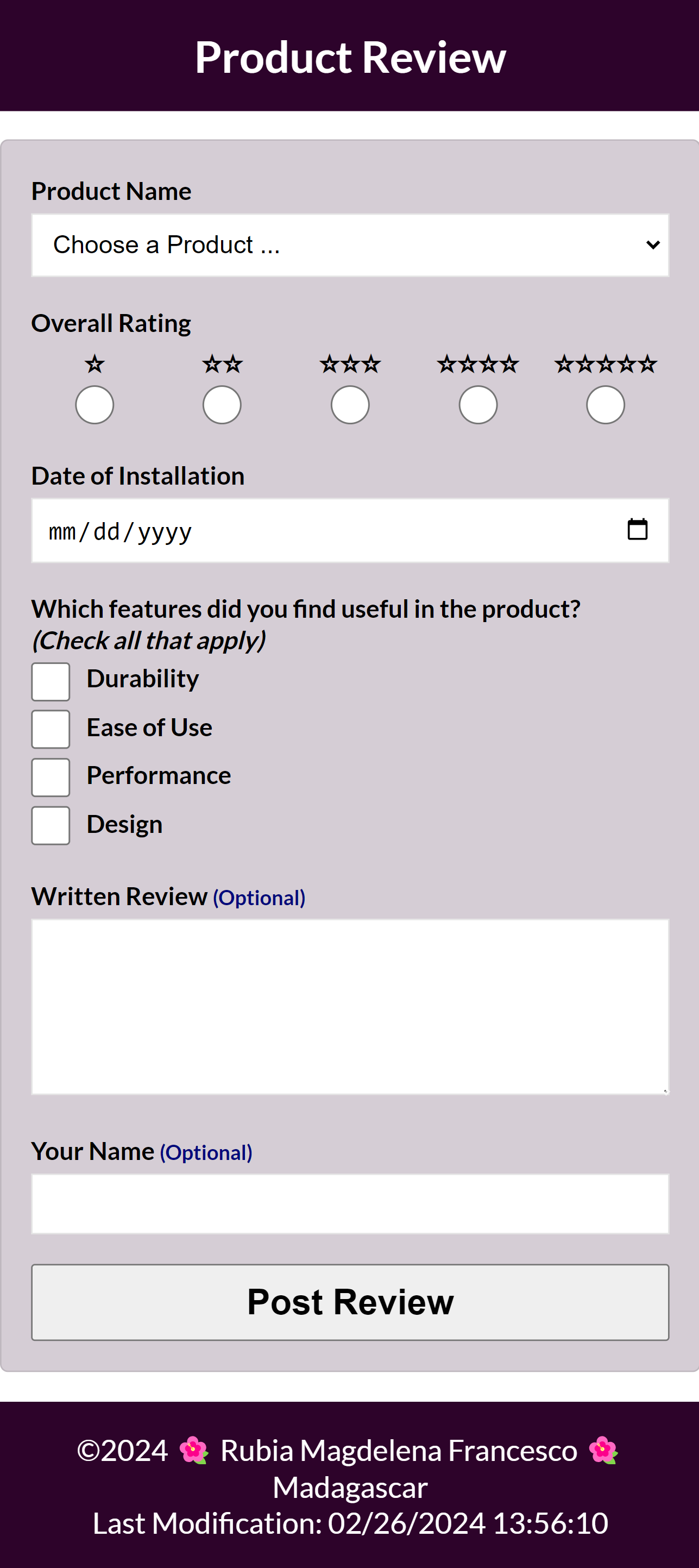
Task
Build an HTML form with a confirmation page. The form must meet the given specifications listed in the instructions. The design is up to you but must meet good form design principles. Here is an example screenshot of what you will be building.
Associated Course Learning Outcomes
- Develop responsive web pages that follow best practices and use valid HTML and CSS.
- Demonstrate proficiency with JavaScript language syntax.
- Use JavaScript to respond to events and dynamically modify HTML.
- Demonstrate the traits of an effective team member including clear communication, collaboration, fulfilling assignments, and meeting deadlines.
Activity Instructions
File and Folder Setup
- Create a new file named "form.html".
- Add supporting CSS files and JavaScript files as needed and place them into their appropriate folders.
HTML
- Include the standard HTML head and body components required in this class and include the common footer content used on assignments.
- The HTML form must have the following fields, attributes, and functionality:
-
Form
- The
form
itself should use amethod
of "get" in order to be supported on GitHub. - The
action
should reference a form confirmation page named "review.html".
- The
-
Product Name
- Use a
select
element with an appropriateid
attribute,name
attribute, and set it to berequired
. - The first
option
in the select element is an instructional placeholder that says "Select a Product ..." and isdisabled
andselected
so that it displays by default but cannot be clicked by the user. - The remaining
option
s are created dynamically using a provided product array.
Each option must have avalue
attribute that is the product name.A product array of objects is provided below to help you understand how to build a select field with the dynamic options. Normally the data would come from an external source.
- Use a
-
Overall Rating
- The overall product rating should have five levels (1 to 5) or stars.
The example form uses star entities ☆ (☆) to display the level. You are free to employ a design of your choice. For example, here is another example of using stars that fill up on selection: Form Input Radio Star+ – CodePen.
- Use a
input
of typeradio
for each of the levels. - The required attributes are an
id
,name
(each of the radio buttons should have the same name value), andrequired
Why should each of the radio buttons that are part of this rating have the same
name
value?
- The overall product rating should have five levels (1 to 5) or stars.
-
Date of Installation
- Use a
input
of typedate
to allow the user to select the date the product was installed. - The required attributes are an
id
,name
, andrequired
- Use a
-
Useful Features
- This field allows the user to select all the listed features that they found useful.
This is a check all that apply field. - Use a
input
of typecheckbox
- Each checkbox should have an
id
,name
, andvalue
attribute.
- This field allows the user to select all the listed features that they found useful.
-
Written Review
- Use a
textarea
element to allow the user to write a review. - The required attributes are an
id
andname
- The written review is not required by the user.
- Use a
-
User Name
- Use a
input
of typetext
for the user to enter their name. - Add the
id
andname
attributes. - The user name entry is optional.
- Use a
-
Form Submission Button
- Use a
input
of typesubmit
with an appropriatevalue
that indicates the form action purpose.
- Use a
-
Form
- Each form field must have an associated
label
. - Check that the keyboard tab order is correct.
- Include the common footer content found on all assignments.
CSS
- Use your own color schema and typography choices.
You are responsible to practice good design principles of alignment, color contrast, proximity, repetition, and usability in all of your work.
- Practice good design by laying out the form according to the principles you were taught in the HTML forms learning activity. You are responsible to create a visually appealing design that adheres to design principles that support usability and an overall positive user experience.
JavaScript
- Use JavaScript to populate the Product Name options where the array's
name
field is used for theselect
option
display and the array'sid
is used for thevalue
field.
Copy the following array of product objects into your JavaScript file to use as the data source.Product Array
const products = [ { id: "fc-1888", name: "flux capacitor", averagerating: 4.5 }, { id: "fc-2050", name: "power laces", averagerating: 4.7 }, { id: "fs-1987", name: "time circuits", averagerating: 3.5 }, { id: "ac-2000", name: "low voltage reactor", averagerating: 3.9 }, { id: "jj-1969", name: "warp equalizer", averagerating: 5.0 } ];
- Use localStorage to keep track of the number of reviews completed by the user client by adding a counter to the form submission review.html page. Every time that page is loaded successfully upon form submission, add one to the counter.
Testing
- Continuously check your work by rendering the page locally using Live/Five Server in VS Code.
- Use the browser's DevTools to check for JavaScript runtime errors in the console or click the red, error icon in the upper right corner of DevTools.
- Use DevTools CSS Overview to check your color contrast.
- Generate the DevTools Lighthouse report and run diagnostics for
Accessibility, Best Practices, and SEO in both the mobile and
desktop views.
It is best to test your page in a Private or Incognito browser window.
Submission and Audit
- Commit your local repository and push or upload your work to your GitHub Pages enabled wdd 131 repository on GitHub.
- Use this ✔ Page Audit Tool to self-check your work for some required HTML elements and CSS content. This audit tool is also used by the assessment team.
- Share your work by posting your URL in the course's Microsoft Teams weekly forum and comment on your peers' work by providing constructive feedback.
- Return to Canvas and submit your correct GitHub Pages enabled URL.
https://your-github-username.github.io/wdd131/form.html