W02 Learning Activity: Document Object Model
Overview
A key skill for any frontend web developer is the ability to manipulate DOM (DOM), a JavaScript object that is created after the browser initially renders the document. Manipulating the DOM means to read, edit, update, or delete the document, including CSS properties, dynamically. Essentially, the DOM is the outline of the HTML and content nodes.
The purpose of this activity is to introduce the HTML DOM and to learn how to manipulate the document using JavaScript.
Associated Course Learning Outcomes
Demonstrate proficiency with JavaScript language syntax.
Use JavaScript
to respond to events and dynamically modify HTML.
Prepare
- Reference: Manipulating documents – MDN.
Note that the Active learning: A dynamic shopping list example found at the end of the MDN article will help you complete this week's activities.
- Practice: Some common DOM manipulation concepts/use cases.
- To select an HTML element from the document using the querySelector method.
This line of code selects the first instance of an article element from the document and assigns the element as a reference to the variable namedarticle
.const article = document.querySelector('article');
- To change the innerHTML property of an existing element.
This line of code uses an existing variable that has already selected an element and changes its innerHTML property value.article.innerHTML = 'innerHTML supports <strong>HTML</strong> tags. The textContent property does not.';
- To change the inline CSS style of an element.
This line of code changes the text-align CSS property of the selected element.article.style.textAlign = 'right';
- To change an attribute of an element.
This line of code adds and attribute class to the element and gives it a value.article.setAttribute('class', 'active');
An alternative way to change an attribute of an element—specifically the class attribute—is by directly manipulating the element's
classList
.
This method is often preferred when working with class manipulation because classList provides additional methods like add, remove, toggle, and contains, making it more convenient and expressive for managing classes on an element.articleElement.classList.add('active');
- To create an element.
This line of code creates a new <p> element and stores it in the variable named paragraph.const paragraph = document.createElement('p');
- To append an element with additional content or elements.
These lines of code add content to the end of the article element.article.appendChild(paragraph); article.append(paragraph, 'Hello World Addition!');
The append() method allows you to include multiple arguments to append to the element in the specified order.
- To remove an element from the document.
These lines of code remove the paragraph from the article and then, the article itself.article.removeChild(paragraph); article.remove();
- To select an HTML element from the document using the querySelector method.
Activity Instructions
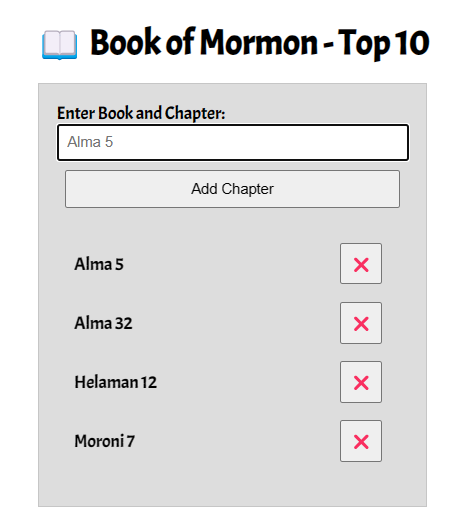
This Book of Mormon application will be added upon in future learning activities. We start now with the interface and basic DOM assignments and build.
This app allows users to enter their favorite Book of Mormon chapters and display them on a list that is updated automatically on the screen. Entries can then be deleted from the displayed list.
- Create an HTML file named "bom.html" in the week02 folder.
- Your bom.html HTML document should include the basic meta tags and an appropriate title.
- Create an external CSS file and a JS file and place them in appropriate subfolders within
the week02 folder.
Check Your Understanding
In the week02 folder, create two folders named styles and scripts. Your CSS file will go in the styles folder and your JavaScript file will go in the scripts folder. You already have folders on the root wdd131 named styles and scripts but that is OK. We will treat this week02 folder independently of the outer wdd131 root folder.
- Copy and paste the basic interface (the HTML and CSS) from the following CodePen ☼ – BOM Top 10.
- In your blank js file, declare three (3) variables that hold references to the
input, button, and list elements.
Check Your Understanding
const input = document.querySelector('#favchap'); const button = document.querySelector('button'); const list = document.querySelector('______'); // you need to fill in the blank to reference the HTML element that is a unordered list element.
- Create a
li
element that will hold each entry's chapter title and an associated delete button.Check Your Understanding
const li = document.createElement('li');
We identified this variable as li, however, that was just for simplicity.
The variable identifier/name did not have to be named the same as the element being created. - Create a delete
button
.Check Your Understanding
const deleteButton = document.createElement('button');
- Populate the
li
element variable'stextContent
orinnerHTML
with the input value.Check Your Understanding
li.textContent = input.value;
textContent is preferred over innerHTML because it is more secure.
However, if you need to include HTML tags, then you would use innerHTML.
textContent will not render HTML tags. It will display the tags as text.Why is the value property used?
Because the input variable references an HTML input text field and that is what is wanted, i.e., the user's entry. Here is the HTML that was provided:<input type="text" id="favchap" placeholder="Alma 5">
- Populate the button textContent with a ❌.
Check Your Understanding
deleteButton.textContent = '❌';
- Append the
li
element variable with the delete button.Check Your Understanding
li.append(deleteButton);
- Append the
li
element variable to the unordered list in your HTML.Check Your Understanding
list.append(li);
So far, You have setup the interface and completed some basic DOM interaction. The application will not work at this point. The next activity will teach how to respond to events, like button clicks. You also need to wait for the user to make an entry into the favorite chapter text field before processing.
You need to consider screen readers and how they will interpret anything in the content. For example, the delete button just has an emoticon and may not read correctly as the button to remove a chapter. What can we do? One solution is to create a aria-label attribute on the button with a value like "Remove Alma 5".
Submission
- Continuously save your work. We will add additional functionality in the next activity.