HTML: The Form Element
Overview
Forms allow us to interact with the user and collect data. The form element is the container for all form elements and includes attributes that enable data processing. In this activity, you will only focus on the frontend design and development, learning how to build a form using basic form elements. Processing the form data after a submission is beyond the scope of this course.
"Web forms are one of the main points of interaction between a user and a website or application. Forms allow users to enter data, which is generally sent to a web server for processing and storage, or used on the client-side to immediately update the interface in some way." - MDN
Prepare
- Read the following sections from the article "Best
Practices for Form Design":
- The components of a form
- Form structure
- Input fields
- Read and Try It Yourself: HTML Forms - w3schools.com
- Read: "Help users enter data in forms"
by Google Chrome Developer web.dev.
This article provides a helpful overview of the form element and its attributes, which you will use in this activity.
Activity Instructions
Getting Started
- In the "week05" folder in your wdd130 course repository, create a new file named "poll-form.html".
- Create a basic HTML page with a
header
,main
, andfooter
.Check Your Understanding
<!DOCTYPE html> <html lang="en-us"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>WDD 130 | HTML Form - User Poll</title> </head> <body> <header> <h1>WDD 130 | Your Name | HTML Form - User Poll</h1> </header> <main> </main> <footer> <p>©2025 | HTML Form - User Poll Activity | Your Name</p> </footer> </body> </html>
Build the Form
- Create a
form
element within themain
element. - Within the
form
, include two (2)fieldset
elements withlegend
child elements as shown in this example:<main> <form> <fieldset> <legend>Contact Information</legend> <!-- form elements will go here --> </fieldset> <fieldset> <legend>Questions</legend> <!-- form elements will go here --> </fieldset> </form> </main>
- Add the following input content to the first, "Contact Information"
fieldset
.
Be sure to use thelabel
element for each form element.- User full name using
input
of typetext
, id of"fullname"
and name of"fullname"
- User email address using
input
of typeemail
, an id of"useremail"
, and a name property value of"useremail"
Check Your Understanding
<form> <fieldset> <legend>Contact Information</legend> <label for="fullname">Full Name:</label> <input type="text" id="fullname" name="fullname"> <label for="useremail">Email:</label> <input type="email" id="useremail" name="useremail"> </fieldset> </form>
Another valid way to write this is to surround the input elements with the label element. This is called the "implicit label" method. The following is an example of this method:
<fieldset> <legend>Contact Information</legend> <label>Full Name: <input type="text" name="fullname"></label> <label>Email: <input type="email" name="useremail"></label> </fieldset>
- User full name using
- Add the following input content to the second, "Questions"
fieldset
.
Be sure to use alabel
element for each form element.- "What is your favorite browser?"
Use aselect
element with three or moreoptions
and be sure to includevalues
. Provide aname
andid
attribute and value of"browser"
. - "Do you have a household pet?"
Use a singlecheckbox
element. Theid
andname
attribute value is"pet"
. - "List the places you have lived."
Use atextarea
element and provideplaceholder
attribute values. In addition, provide aname
andid
attribute value of"feedback"
and set the number ofrows
.
Check Your Understanding
<fieldset> <legend>Questions</legend> <label for="browser">What is your favorite internet browser?</label> <select id="browser" name="browser"> <option value="chrome">Chrome</option> <option value="firefox">Firefox</option> <option value="safari">Safari</option> <option value="edge">Edge</option> <option value="brave">Brave</option> <option value="other">Other</option> </select> <label for="pet">Do you have a household pet?</label> <input type="checkbox" id="pet" name="pet"> <label for="feedback">List the places you have lived:</label> <textarea id="feedback" name="feedback" rows="4" placeholder="Stillwater Florida, Amsterdam Netherlands, Tibuktu Mali"></textarea> </fieldset>
- "What is your favorite browser?"
- Add a
button
with a type ofsubmit
at the end of the form element. The text content of the button is"Submit Poll"
.Check Your Understanding
<button type="submit">Submit Poll</button>
Where should thisbutton
element be placed in the HTML document?Hint:
Make sure it is within the
form
element.
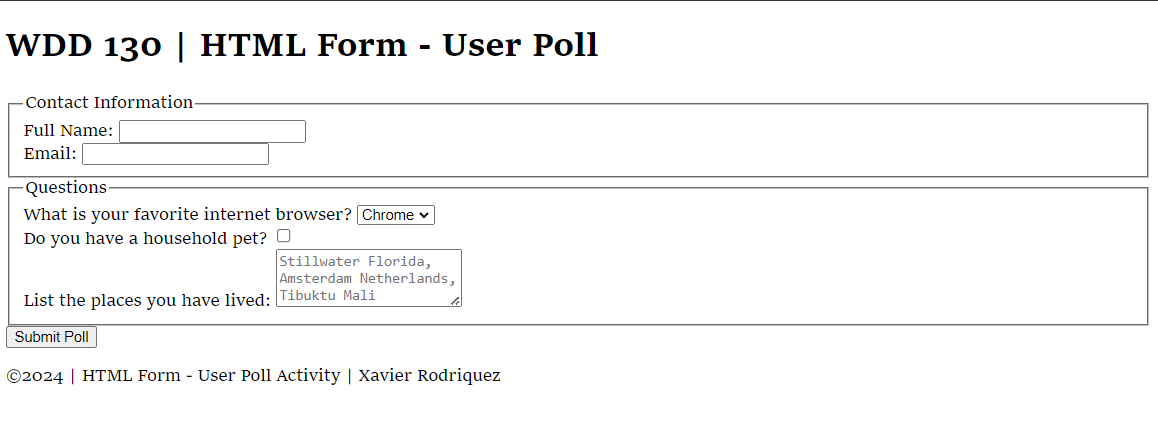
Testing and Submission
- Test and validate your HTML in the browser.
- Save your work. CSS styling will be added in the next activity.
Note that this form does not include processing the user input. We are only working with the frontend of the form design and structure. This scope of this course does not include the processing of the data.