W03 Code: Sets and Maps
This assignment must be completed individually to ensure you are meeting all course outcomes. You should not complete this assignment within a group. If you obtain help from a tutor, the tutor should help you understand principles but should not help you answer these problems. It is an honor code violation to obtain answers for these problems from others including using the internet (i.e. sites that allow students to share their solutions).
Running and Debugging
-
You should not edit any files that have the comment
DO NOT MODIFY THIS FILE
at the top of them. Any edits you make to these files will be removed before grading. - Running all tests for this assignment: Click on Run and Debug in the sidebar, then select this week's code assignment from the drop-down at the top. Click the green Start Debugging button next to the drop-down. The integrated terminal will show the files being compiled and then the tests being run. It will print a summary of which tests passed and failed.
-
Running individual tests: Navigate to the test file ending in
_Tests.cs
in the week's code directory. Visual Studio Code will show inline buttons to Run Test for each test method, or to Run All Tests for each test class. -
Debugging individual tests: Navigate to the test file ending in
_Tests.cs
in the week's code directory. Visual Studio Code will show inline buttons to Debug Test for each test method, or to Debug All Tests for each test class.- If you put a breakpoint inside your code or inside the test, the only way to hit it will be by clicking Debug Test or Debug All Tests in this file.
-
If you want to debug by printing output to the terminal, you must use
Debug.WriteLine()
to do so. Using that may require you to addusing System.Diagnostics;
to the top of the file. UsingConsole.WriteLine()
, as is done in other parts of the template project, will not correctly print output to the console when a test is running. Any output generated withDebug.WriteLine()
will appear in the Debug Console portion of the integrated terminal when clicking Debug Test or Debug All Tests in this file.
Instructions
This coding assignment contains five separate problems. To receive full credit on this assignment, you must successfully complete any four of the five problems. If you complete all five problems you are eligible to receive extra credit.
All of the files needed for this assignment can be found in the week03/code
folder in the course repository. The start of each problem can be found in the SetsAndMaps.cs
or Maze.cs
code files.
Find Pairs with Sets
- Assume you have been asked in an interview to describe how you would find symmetric pairs of two letter words in a list in O(n) time using a set. You should assume that the the words are lower case, two characters long, and contain no duplicates. As an example, if the word list was
[am, at, ma, if, fi]
then the result would be["am & ma", "if & fi"]
. The order of the array would not matter. Note that theat
would not be returned becauseta
was not in the list. As a special case, if the letters are the same (example:aa
) then it would not match anything else (remember the assumption above that there were no duplicates) and therefore should not be returned. - Implement the
FindPairs
function with O(n) time using sets.
Degree Summary
Finish implementing the SummarizeDegrees
function in the SetsAndMaps
class which reads a file and creates a dictionary containing a summary of all the degrees (education) that people earned as reported in the file. The degree can be found in column 4 of the file. Your code should automatically determine the degree names. You should use the following file for testing: census.txt
.
Anagrams
Finish implementing the IsAnagram
function to determine if two words are anagrams. Two words are an anagram if they use the same letters and the same number of letters. For example, "CAT" and "ACT" are anagrams but "DOG" and "GOOD" are not because "GOOD" has two O's. You must use a dictionary to solve this problem. It is important to note the following assumptions:
- When determining if two words are anagrams, you should ignore any spaces.
- You should ignore letter case. For example, 'Ab' and 'bA' should be considered anagrams.
Reminder: You can access a letter by index in a string by using the myString[3]
notation.
Maze
Implement the MoveLeft
, MoveRight
, MoveUp
, and MoveDown
functions in the Maze
class. The code provides a sample Maze in the form of a dictionary where the key represents the (x,y) coordinate in the maze and the value contains the valid movements in the format (left, right, up, down). The valid movements are set to true
and false
. The dictionary maze can be represented graphically as follows (note that it is assumed there is a wall going around the entire maze which means that you cannot leave the outside boundaries of the maze):
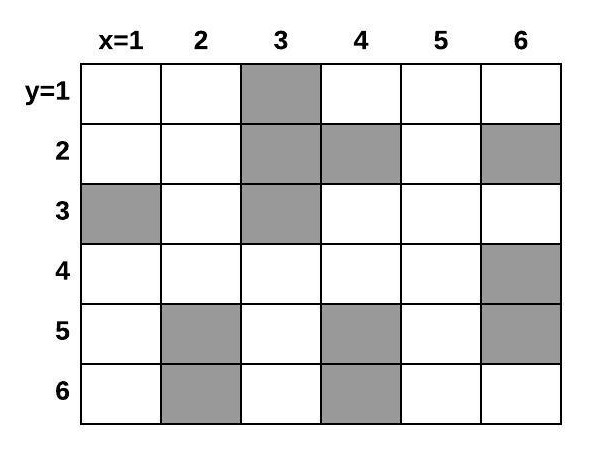
Earthquake JSON Data
Finish implementing the EarthquakeDailySummary
function to return an array of formatted strings of all the earthquake locations ('place' attribute) and magnitudes ('mag' attribute) for the current day from the United States Geological Service (USGS) website. The USGS Website provides details about how to interpret the data that you will receive from their server. The data uses JSON (JavaScript Object Notation) format. In C#, JSON data can be deserialized into an object. Objects are essentially a map, where the keys are the property names and the values are the property values. The difference between objects and dictionaries is that objects are defined at compile time, where dictionaries can be added to dynamically throughout the program execution.
Examine the data that you will be deserializing and use it to populate the FeatureCollection
object with the right properties. You will need to create custom classes to map the data appropriately. See the reading for an example of how to interact with JSON in C#.
Each string in the returned array should be formatted to display the data like this, however the places and magnitudes of the earthquakes will be different as this data changes every day.
[
1km NE of Pahala, Hawaii - Mag 2.36,
58km NW of Kandrian, Papua New Guinea - Mag 4.5,
16km NNW of Truckee, California - Mag 0.7,
9km S of Idyllwild, CA - Mag 0.25,
14km SW of Searles Valley, CA - Mag 0.36,
4km SW of Volcano, Hawaii - Mag 1.99,
...
]
Grading
To receive full credit on this assignment, you must successfully complete any four of the five problems. If you complete all five problems you are eligible to receive extra credit.
Submission
When you have finished the assignment:
- Make sure that all of your changes are committed to your course repository.
- Push your latest changes to GitHub.
- Return to Canvas to submit a link to your GitHub repository.
Other Links:
- Return to: Week Overview | Course Home