W05: Programming Tasks
Instructions
Setup
- Download the starter files (2). Place the
w05-task.html
file on the rootcse121b
folder and thew05-task.js
file in thescripts
folder.
Review the Public Temple Data
- Navigate to this temple JSON file by clicking on the link. Your browser will handle the parsing of the data to make it readable.
- Review the temple data format and naming structure.
Complete the following steps in the w05-task.js file. Do not alter the
w05-task.html file.
Declare Global Variables
- Declare a
const
variable namedtemplesElement
that references the HTML div element that eventually will be populated with temple data. - Declare a global empty array variable to store a list of temples named
templeList
.
Function: displayTemples()
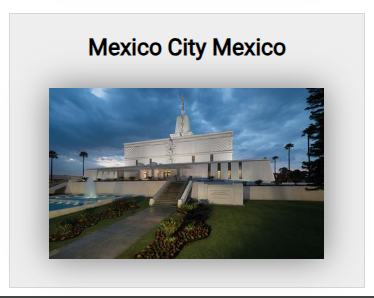
This function will build a temple display "card" for each item in the temple list passed to the function.
-
Declare a function expression using
const
nameddisplayTemples
that uses an arrow function to accept a list of temples as an array argument.Check Your Understanding
const displayTemples = (temples) => {
- Process each temple in the temple array using a forEach method and do the
following for each temple item:
- Create an HTML
<article>
element (createElement
). - Create an HTML
<h3>
element and add the temple'stempleName
property to this new element. - Create an HTML
<img>
element and add the temple'simageUrl
property to thesrc
attribute and the temple'slocation
property to thealt
attribute. - Append the
<h3>
element and the<img>
element to the<article>
element as children. (appendChild
) - Append the
<article>
element to the globaltemplesElement
variable declared in Step 2.Check Your Understanding
templesElement.appendChild(article);
- Create an HTML
Function: getTemples()
Get JSON temple data using fetch().
- Create another function expression called
getTemples
. Make it anasync
anonymous, arrow function.Check Your Understanding
const getTemples = async () => {
- In the function, declare a
const
variable namedresponse
thatawait
s the built-infetch
method calling the temple data, absolute URL given in Step 2 above.Check Your Understanding
const response = await fetch("https://byui-cse.github.io/cse121b-ww-course/resources/temples.json");
- Convert your fetch response into a JavaScript object (
.json
) and assign the result to thetempleList
global array variable you declared in Step 3 above. Make sure the the execution of the code waits here as well until it finishes.💡If you are stuck with any of these steps, consider using the learning activity materials provided. - The last statement in the
getTemples
function calls thedisplayTemples
function and passes it the list of temples (templeList
).💡A good idea here is to test your code by calling thegetTemples
function at the end of your JavaScript file. This will make sure that your code works before you move on to the next step. You canconsole.log(templeList)
to review the results.
Function: reset()
Clear the displayed list of temples.
- Declare a function expression named
reset
that clears all of the<article>
elements from thetemplesElement
.
Function: filterTemples()
Sort the list of temples by the selected value of the HTML select
element with an ID of filtered
. The preset selections include temples located in
the state of Utah, those outside the state of Utah, and temples built before 1950 on the list.
- Declare a function expression named
filterTemples
. - The function should accept a argument in a parameter named
temples
. - In this function, first call the
reset
function to clear the output. - Define a variable named
filter
that obtains thevalue
of the HTML element with the ID offiltered
(The pull-down menu). - Use a
switch
statement that uses thefilter
value as the selector responding to four (4) cases. - For each case, call the
displayTemples
function using an filter statement that filters thetemples
parameter for the four options provided.- "utah": filter for temples where the
location
contains "Utah" as a string. - "notutah": filter for temples where the
location
does not contain "Utah" as a string. - "older": filter for temples where the
dedicated
date is before 1950. (compare versusnew Date(1950, 0, 1)
). - "all": no filter. Just use
temples as the argument.
- "utah": filter for temples where the
Event Listener: filterTemples Element change
- Add a
change
event listener to the HTML element with an ID offiltered
that calls thefilterTemples
function and sends a arrow function result with thetempleList
as the argument.Check Your Understanding
document.querySelector("#filtered").addEventListener("change", () => { filterTemples(templeList) });
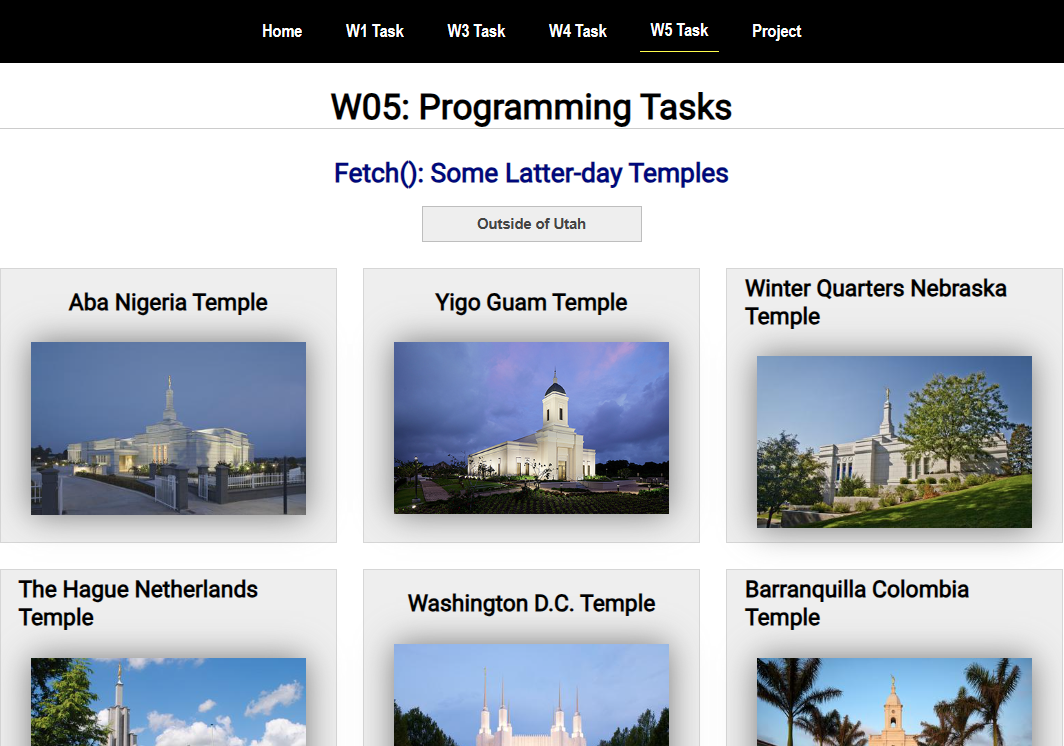
Testing
- Verify that you are calling
getTemples()
at the bottom of the JavaScript file. - Test your work by using Live Server and debug as needed.
Submission
- Commit and push your work to GitHub.
- Submit the GitHub Pages rendered URL in Canvas.
💡Here is an example of what the URL should look like:
https://yourgithubusername.github.io/cse121b/w05-task.html
or
https://yourgithubusername.github.io/cse121b
is OK because each assignment is already included in the provided navigation structure on this home page for the course.
Stretch
Add additional options such as sorting the temples alphabetically.