W04: Programming Task
In your previous weeks' tasks, you declared and used individual variables. In this task, you are to rework the home page biography task from week 02 using object literals.
Instructions
Setup
- Download the starter files (2). Place the
w04-task.html
file on the rootcse121b
folder and thew04-task.js
file in thescripts
folder.
Object Literal
Do the following work in the
Do 🚫not alter your index.html file.
w04-task.js
file which is linked to the
w04-task.html
file.Do 🚫not alter your index.html file.
-
Declare a new object literal variable named
myProfile
to hold information about yourself and assign an empty object to the variable.Check Your Understanding
let myProfile = {};
-
Add a property to this object literal named
name
and set its value to be your name as a string.Check Your Understanding
let myProfile = { name: "Patrick Juarez" }
-
Add a property named
photo
. Set its value to be an image's path and name (one used in Programming Task 2) as a string. -
Add a property named
favoriteFoods
. Set its value to be an array of your favorite foods as stringsCheck Your Understanding - Example
favoriteFoods: [ 'Rice', 'Tikka Masala', 'Prioshki', 'Shrimp', 'Banana Cream Pie' ],
-
Add a property named
hobbies
. Set its value to be an array of your hobbies as strings. -
Add a property named
placesLived
. Set its value to be an empty array. - Using code outside of the
myProfile
definition, add an item to theplacesLived
array where this new item itself is object with two properties:place
andlength
and for each of these properties, add appropriate values as strings.Check Your Understanding
// This occurs outside of the object definition. myProfile.placesLived.push( { place: 'San Francisco, CA', length: '1 year' } );
-
Add additional object literals with appropriate values to the
placesLived
array for each place you have lived.
DOM Manipulation (Output)
-
Assign the value of the
name
property of themyProfile
object to the HTML element with an ID ofname
.Check Your Understanding
document.querySelector('#name').textContent = myProfile.name;
-
Assign the value of the
photo
property as thesrc
attribute of the HTML<img>
with an ID ofphoto
. -
Assign the value of the
name
property as thealt
attribute of the HTML<img>
with an ID ofphoto
. -
For each favorite food in the
favoriteFoods
property,💡Use a forEach loop to iterate over thefavoriteFoods
array.- create an HTML
<li>
element (💡createElement
), - place the value of the
favoriteFoods
array element into thetextContent
of this newli
element, and then - append this new
<li>
element with content as a child of the HTML<ul>
element with an ID offavorite-foods
.
Check Your Understanding
💡What is the difference between append method and the appendChild() method when it comes to manipulating the DOM and adding elements to a parent element?myProfile.favoriteFoods.forEach(food => { let li = document.createElement('li'); li.textContent = food; document.querySelector('#favorite-foods').appendChild(li); });
- create an HTML
-
Repeat the previous process of creating a list of items for each hobby in the
hobbies
property of the object and appending each item to the HTML<ul>
element with an ID ofhobbies
. -
For each object in the
placesLived
property:- Create an HTML <dt> element and put its
place
property in the <dt> element. - Create an HTML <dd> element and put its
length
property in the <dd> element
- Create an HTML <dt> element and put its
-
Append the HTML
<dt>
and<dd>
elements created above to the HTML<dl>
element with an ID ofplaces-lived
.
Testing
- Test your work by using Live Server and debug as needed.
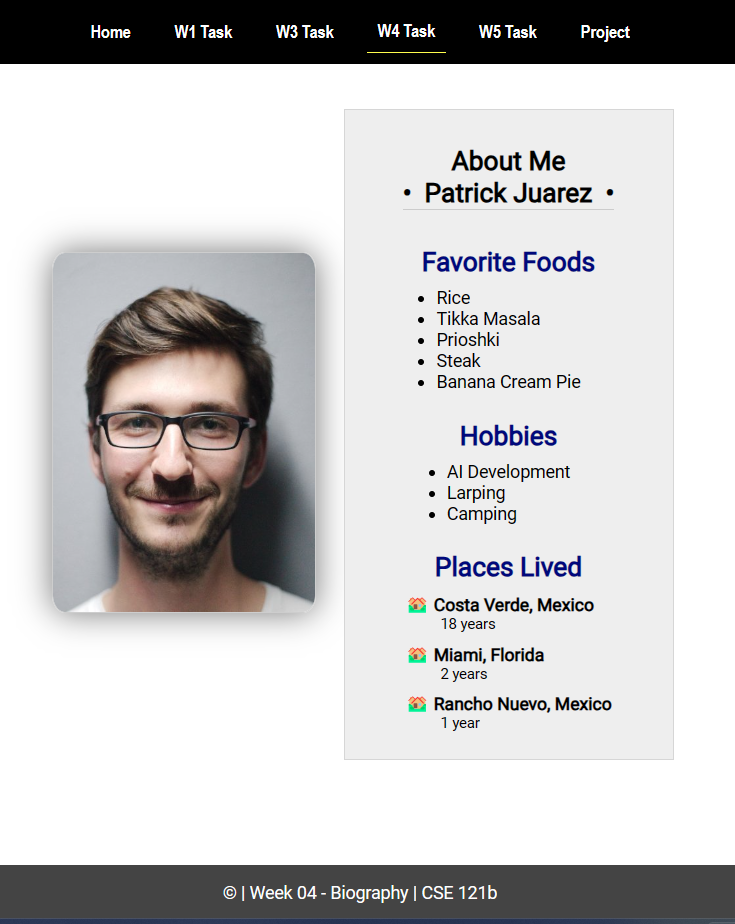
Submission
- Commit and push your programming task work to your cse121b GitHub Pages enabled repo.
- Submit the URL of your rendered page on GitHub to Canvas.
💡Here is an example of what the URL should look like:
https://yourgithubusername.github.io/cse121b/w04-task.html
or
https://yourgithubusername.github.io/cse121b
is OK because each assignment is already included in the provided navigation structure on this home page for the course.