W03: Programming Task
Instructions
Setup
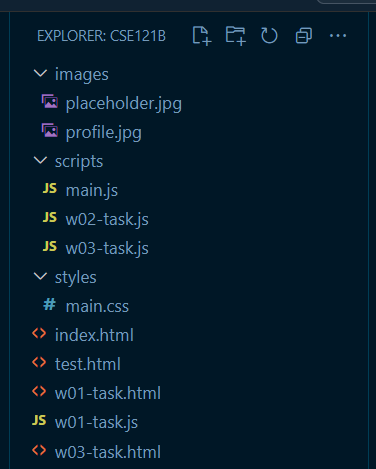
- Download the starter files (2). Place the
w03-task.html
file on the rootcse121b
folder and thew03-task.js
file in thescripts
folder.
Function Declaration
For Steps 2 through 5, use VS Code to edit the
w03-task.js
file.
✔️Addition Feature
- Using function declaration, define a function named
add
that takes two arguments and assigns them to the parametersnumber1
andnumber2
.Check Your Understanding
function add (number1, number2) { // function body }
- In the function body,
return
the sum of the parametersnumber1
andnumber2
.Check Your Understanding
return number1 + number2;
- Using a function declaration, define another function named
addNumbers
that gets the values of two HTML form controls with IDs ofadd1
andadd2
.💡When getting the values of the HTML form controls (e.g.,<input>
), use thevalue
property and go ahead and convert those strings to numbers.Check Your Understanding
let addNumber1 = Number(document.querySelector('#add1').value);
- Next, in the
addNumbers
function, call theadd
function using those two arguments and assign the return value to an HTML form element with an ID ofsum
Check Your Understanding
document.querySelector('#sum').value = add(addNumber1, addNumber2);
- Add a "click" event listener to the HTML button with an ID of
addNumbers
that calls theaddNumbers
function. This line of code is NOT located inside a function. Why❔Check Your Understanding
document.querySelector('#addNumbers').addEventListener('click', addNumbers);
Function Expression
✔️Subtraction Feature
- Using function expressions, repeat the items in Step 2 with new functions
named
subtract
andsubtractNumbers
and HTML form controls with IDs ofsubtract1
,subtract2
,subtractNumbers
, anddifference
.💡You should verify that these are the form control ID values.
Arrow Functions
✔️Multiplication Feature
- Using arrow functions, repeat the items in Step 2 with arrow functions
named
multiply
andmultiplyNumbers
and HTML form controls with IDs offactor1
,factor2
,multiplyNumbers
, andproduct
.
Your Choice
✔️Division Feature
- Using any combination of function declaration types, repeat the items in
Step 2 with new functions named
divide
anddivideNumbers
and HTML form controls with IDs ofdividend
,divisor
,divideNumbers
, andquotient
.Remember that you can view the provided HTML file to see the structure and IDs of the HTML elements on the page to gain reference and context.
Selection Structures
- Add an event listener for the Get Total Due button when clicked that
invokes the following functionality:
- Declare and instantiate a variable that stores the numeric value
entered
by the user in the
subtotal
field.User input validation is not required in this task, however, input validation is best practice. Consider adding it as a stretch goal by checking that the input value is a valid, numeric amount. - Check IF the membership checkbox has been checked by the user to apply a 20% discount to the subtotal amount.
- Output the total to the the
total
span
in the format shown with two decimals using a template string.
- Declare and instantiate a variable that stores the numeric value
entered
by the user in the
Array Methods - Functional Programming
- Declare and instantiate an array variable to hold the numbers 1 through 13.
Check Your Understanding
let numbersArray = [1,2,3,4,5,6,7,8,9,10,11,12,13];
- Assign the value of the array variable to the HTML element with an ID of
array
. - Use the
filter()
array method to find all of the odd numbers of the array variable and assign the result to the HTML element with an ID ofodds
💡Hint: Use the modulus operator%
to find the remainder of dividing a number by 2. If the remainder is 1, the number is odd. If the remainder is 0, the number is even. - Use the
filter()
array method to find all of the even numbers of the array variable and assign the result to the HTML element with an ID ofevens
.Check Your Understanding
document.querySelector('#evens').innerHTML = numbersArray.filter(number => number % 2 === 0);
- Use the
reduce()
array method to sum the array variable elements and assign the result to the HTML element with an ID ofsumOfArray
Check Your Understanding
expression example only
numbersArray.reduce((sum, number) => sum + number)
- Use the
map()
array method to multiple each element in the array variable by 2 and assign the result to the HTML element with an ID ofmultiplied
.Check Your Understanding
expression example only
numbersArray.map(number => number * 2)
- Use the
map()
andreduce()
array methods to sum the array elements after multiplying each element by two. Assign the result to the HTML element with an ID ofsumOfMultiplied
.
Testing
- Test your work by using Live Server and debug as needed.
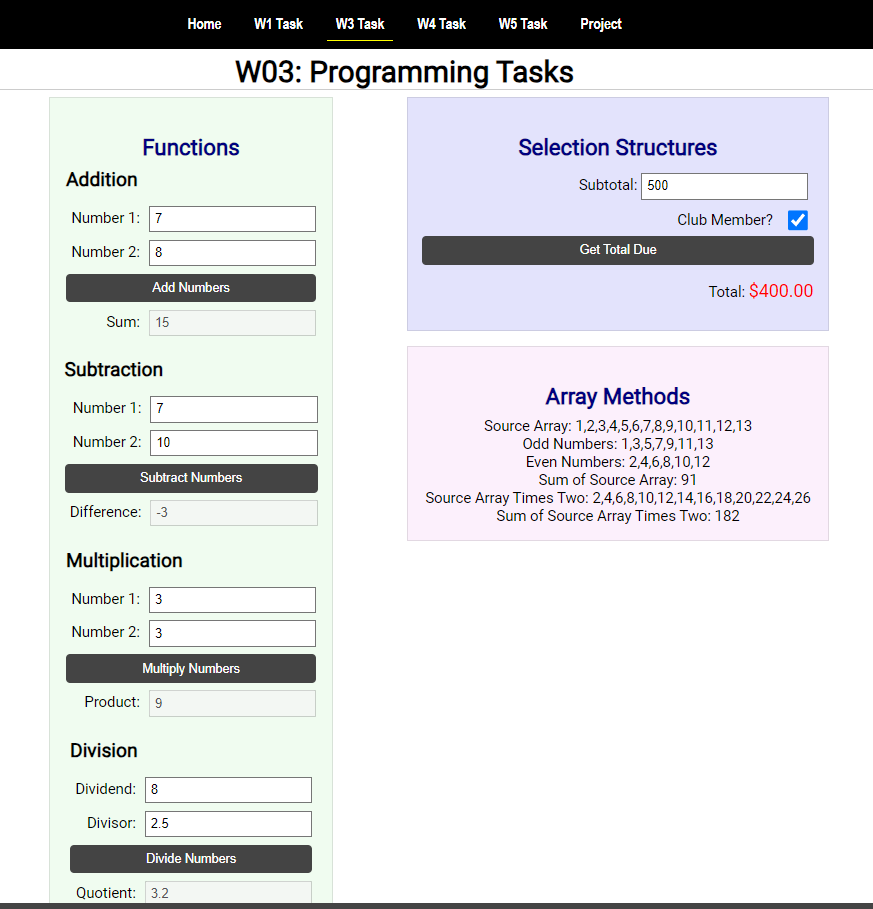
Submission
- Commit and push your work to GitHub.
- Submit the GitHub Pages rendered URL in Canvas.
💡Here is an example of what the URL should look like:
https://yourgithubusername.github.io/cse121b/w03-task.html
or
https://yourgithubusername.github.io/cse121b
is OK because each assignment is already included in the provided navigation structure on this home page for the course.