05 Prove Milestone: Testing and Fixing Functions
Purpose
Prove that you can write a Python program and write and run test functions to help you find and fix mistakes in your program.
Problem Statement
Getting clean water to all buildings in a city is a large and expensive task. Many cities will build an elevated water tank, and install a pump that pushes water up to the tank where the water is stored. In the city, when a thirsty person opens a water faucet, water runs from the tank through pipes to the faucet. Earth’s gravity pulling on the water in the elevated tank pressurizes the water and causes it to spray from the faucet.
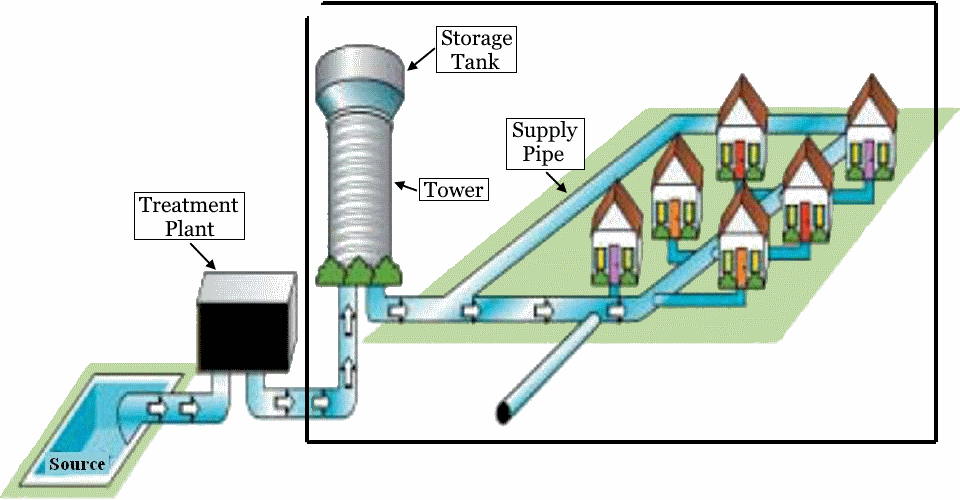
Before a city builds a water distribution system, an engineer must design the system and ensure water will flow to all buildings in the city. An engineer must choose the tower height, pipe type, pipe diameter, and pipe path. Engineers use software to help them make these choices and design a working water distribution system.
Assignment
Write a Python program that could help an engineer design a water distribution system. During this prove milestone, you will write three program functions and three test functions as described in the Steps section below.
Help
If you’re having trouble completing this assignment, reading related online documentation, using a generative AI as a tutor, or working with a human tutor will help you complete it.
Helpful Documentation
- The preparation content for this lesson explains how to use
pytest
,assert
, andapprox
to automatically verify that functions are correct. It also contains an example test function and links to additional documentation aboutpytest
. - The
pytest
approx
function accepts optional named arguments. One of those named arguments is abs. The abs named argument causes theapprox
function to compare the actual and expected values up to a specified digit after the decimal point and to ignore the following digits. For example, the following two lines of code cause pytest to compare the actual number returned frompressure_loss_from_fittings
to −0.306 to only the third digit after the decimal point and to ignore all digits in the actual number after the 6.Notice in the previous example, that the value for abs is 0.001, which causes the approx function to ignore all digits after the third digit after the decimal point.assert pressure_loss_from_fittings(1.75, 5) \ == approx(-0.306, abs=0.001)
- This video about test functions (20 minutes) shows a BYU-Idaho faculty member writing two test functions and using
pytest
to run them.
Help from an AI Tutor
You could use a generative AI as a tutor to help you write and troubleshoot your program. Bro. Lee Barney created a custom Chat GPT named Pythonista that is designed to focus on Python functions, loops, if statements, and related programming concepts. If your program is generating an error, ask Pythonista a question like this:
I'm writing a Python program that computes water pressure within a municipal water system. When I run my program, it causes the following error. Please suggest some mistakes that might cause this error.
(Copy and paste the error message here.)
You could also ask Pythonista a question about one of your functions, like this:
I wrote the following Python function that is supposed to (type a short description here). However, the function isn't (type what it's not doing correctly here). Please help me fix this function.
(Copy and paste your Python function here.)
Help from a Human Tutor
As a BYU-Idaho campus or online student you can get help from a tutor to complete your CSE 111 assignments. Each tutor is a current BYU-Idaho student employed by BYU-Idaho. Meeting with a tutor is free. It will not cost you any money to meet with a tutor. To get help from a tutor, you simply make an appointment and then meet with the tutor. Campus students meet with tutors in the tutoring center. Online students meet with tutors in Zoom. To make an appointment, follow the instructions in the course tutoring guide.
Steps
Do the following:
- Using VS Code, create a new file and save it as
water_flow.py
- Create another new file, save it as
test_water_flow.py
, and copy and paste the following import statements into the file.from pytest import approx import pytest
- In your
water_flow.py
program, write a function namedwater_column_height
that calculates and returns the height of a column of water from a tower height and a tank wall height. The function must have this header:def water_column_height(tower_height, tank_height):
In your function, use the following formula for calculating the water column height.
h = t +where3w4- h is height of the water column
- t is the height of the tower
- w is the height of the walls of the tank that is on top of the tower
- In your
test_water_flow.py
file, write a test function namedtest_water_column_height
. This test function must callwater_column_height
at least four times to verify that it is working correctly. Use the following numbers in your test function.Tower
HeightTank Wall
HeightExpected Water
Column Height0.0 0.0 0.0 0.0 10.0 7.5 25.0 0.0 25.0 48.3 12.8 57.9 - In your
water_flow.py
program, write a function namedpressure_gain_from_water_height
that calculates and returns the pressure caused by Earth’s gravity pulling on the water stored in an elevated tank. The function must have this header:def pressure_gain_from_water_height(height):
In your function, use the following formula for calculating pressure caused by Earth’s gravity.
P =whereρgh1000- P is the pressure in kilopascals
- ρ is the density of water (998.2 kilogram / meter3)
- g is the acceleration from Earths gravity (9.80665 meter / second2)
- h is the height of the water column in meters
- In your
test_water_flow.py
file, write a test function namedtest_pressure_gain_from_water_height
. This test function must callpressure_gain_from_water_height
at least three times to verify that it is working correctly. Use the following numbers in your test function.Height Expected
Pressureapprox
Absolute
Tolerance0.0 0.000 0.001 30.2 295.628 0.001 50.0 489.450 0.001 - In your
water_flow.py
program, write a function namedpressure_loss_from_pipe
that calculates and returns the water pressure lost because of the friction between the water and the walls of a pipe that it flows through. The function must have this header:def pressure_loss_from_pipe(pipe_diameter, pipe_length, friction_factor, fluid_velocity):
In your function, use the following formula for calculating pressure loss from friction within a pipe.
P =where− f L ρ v22000 d- P is the lost pressure in kilopascals
- f is the pipe’s friction factor
- L is the length of the pipe in meters
- ρ is the density of water (998.2 kilogram / meter3)
- v is the velocity of the water flowing through the pipe in meters / second
- d is the diameter of the pipe in meters
- In your
test_water_flow.py
file, write a test function namedtest_pressure_loss_from_pipe
. This test function must call
pressure_loss_from_pipe
at least seven times to verify that it is working correctly. Use the following numbers in your test function.Pipe
DiameterPipe
LengthFriction
FactorFluid
VelocityExpected
Pressure
Lossapprox
Absolute
Tolerance0.048692 0.00 0.018 1.75 0.000 0.001 0.048692 200.00 0.000 1.75 0.000 0.001 0.048692 200.00 0.018 0.00 0.000 0.001 0.048692 200.00 0.018 1.75 -113.008 0.001 0.048692 200.00 0.018 1.65 -100.462 0.001 0.286870 1000.00 0.013 1.65 -61.576 0.001 0.286870 1800.75 0.013 1.65 -110.884 0.001 - Copy and paste the following code at the bottom of your
test_water_flow.py
file.# Call the main function that is part of pytest so that the # computer will execute the test functions in this file. pytest.main(["-v", "--tb=line", "-rN", __file__])
Testing Procedure
Verify that your functions work correctly by following each step in this procedure:
- Run your
test_water_flow.py
file and ensure that all three of the test functions pass. If any of the test functions don’t pass, there is a mistake in either the program function that you wrote or the test function that you wrote. Read the output frompytest
, fix the mistake, and run thetest_water_flow.py
file again until all the test functions pass.> python test_water_flow.py ======================== test session starts ========================== platform win32 -- Python 3.11.1, pytest-7.2.1, pluggy-1.0.0 -- rootdir: C:\Users\cse111\lesson05 collected 3 items test_water_flow.py::test_water_column_height PASSED [ 33%] test_water_flow.py::test_pressure_gain_from_water_height PASSED [ 66%] test_water_flow.py::test_pressure_loss_from_pipe PASSED [100%] ========================= 3 passed in 0.07s ===========================
Ponder
During this assignment, you wrote three program functions named
water_column_height
,
pressure_gain_from_water_height
, and
pressure_loss_from_pipe
. Also, in a separate file, you
wrote three test functions. Each of the test functions called one of
the program functions multiple times and automatically verified that
the value returned from the program function was correct. If you
worked as a software developer on a large project with four other
software developers, how would test functions help you and the other
developers ensure that your program worked correctly?
Submission
On or before the due date, return to I‑Learn and report your progress on this milestone.