04 Checkpoint: Variable Scope
Purpose
Check your understanding of parameters, arguments, and local variable scope by fixing a program that won’t run because the programmer tried to use variables that were defined in a different function.
Helpful Documentation
- The preparation content for lesson 3 explains what a parameter is.
- The preparation content for lesson 2 explains what an argument is.
- The preparation content for this lesson contains an example program with a mistake similar to the mistake that is in the example program below.
Problem Statement
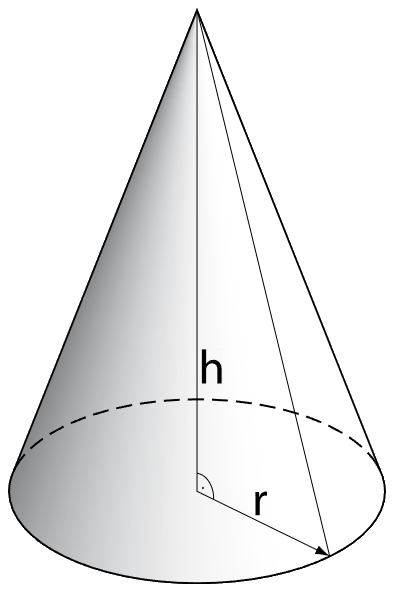
The following example Python program is supposed to ask the user
for the radius and height of a right circular cone and then compute
and print the volume of that cone. The code is almost correct, but
it contains a common mistake. The programmer who wrote it assumed
that the cone_volume
function could use the
radius and height variables that are defined
in the main
function. Of course, this assumption is
incorrect because as the
preparation content for
this lesson explains, variables defined inside a function have local
scope and can be used only inside their containing function.
"""Compute and print the volume of a right circular cone.""" # Import the standard math module so that # math.pi can be used in this program. import math def main(): # Call the cone_volume function to compute # the volume of an example cone. ex_radius = 2.8 ex_height = 3.2 ex_vol = cone_volume() # Print several lines that describe this program. print("This program computes the volume of a right") print("circular cone. For example, if the radius of a") print(f"cone is {ex_radius} and the height is {ex_height}") print(f"then the volume is {ex_vol:.1f}") print() # Get the radius and height of the cone from the user. radius = float(input("Please enter the radius of the cone: ")) height = float(input("Please enter the height of the cone: ")) # Call the cone_volume function to compute the volume # for the radius and height that came from the user. vol = cone_volume() # Print the radius, height, and # volume for the user to see. print(f"Radius: {radius}") print(f"Height: {height}") print(f"Volume: {vol:.1f}") def cone_volume(): """Compute and return the volume of a right circular cone.""" volume = math.pi * radius**2 * height / 3 return volume # Start this program by # calling the main function. main()
Assignment
Do the following:
- Using VS Code, open a new file. Copy and paste all of the example program above into the new file. Save the new file as
cone_volume.py
- Run your
cone_volume.py
program. What error message do you see in the terminal frame? - Fix the
cone_volume
function which begins on line 37 by adding two parameters to its header. What parameter names should you use?
Hint: the parameter names at line 37 and the variable names at line 39 must be the same. - Fix the call to the
cone_volume
function that is inmain
at line 13 by adding two arguments. Because thecone_volume
function has two parameters (you added the two parameters in the previous step), each call to thecone_volume
function must have two arguments. What variable names should you use for the arguments?
Hint: the only variables that you can use must be defined insidemain
above line 13. - Fix the call to the
cone_volume
function that is inmain
at line 28 by adding two arguments. What variable names should you use for the arguments?
Hint: the only variables that you can use must be defined insidemain
above line 28. - Save your program and run it again. Did it run correctly? If not, fix the mistakes until it runs correctly.
Testing Procedure
Verify that your program works correctly by following each step in this testing procedure:
- After you finish the steps of this assignment, run your program and enter the inputs shown below. Ensure that your program’s output matches the output below.
> python cone_volume.py This program computes the volume of a right circular cone. For example, if the radius of a cone is 2.8 and the height is 3.2, then the volume is 26.3 Please enter the radius of the cone: 5 Please enter the height of the cone: 8.2 Radius: 5.0 Height: 8.2 Volume: 214.7
Sample Solution
When your program is finished, compare your program to the sample solution for this assignment. Before looking at the sample solution, you should work to complete this checkpoint program. However, if you have worked on it for at least an hour and are still not finished, feel free to use the sample solution to help you finish your program.
Ponder
The original program that you copied and pasted to start this
assignment didn’t work because the programmer tried to use the
radius and height variables inside the
cone_volume
function. However, the radius
and height variables are defined inside the
main
function and therefore have local scope and cannot
be used outside of main
. To fix the program, you added
two parameters to the cone_volume
function and then
added two arguments to each call of the cone_volume
function.
Why was local variable scope invented by computer scientists? How does local variable scope make a program easier to write and understand?
Submission
When complete, report your progress in the associated I‑Learn quiz.